Question:
How to send Email in Laravel using gmail?Solution:
So, we want to send an email to others or to admins or any email and that is in Laravel. Laravel use SwiftMailer Library to send email and for us that is a pretty nice and simple work.First Step:
We want to send email through gmail. So, we need to setup the gmail account, common sense. Go to gmail account > Sign-in & security > App Password > Generate an app password > Copy the 16 digit password. In that page select mail and select windows computer and generate an app password, See the image after generating an app password.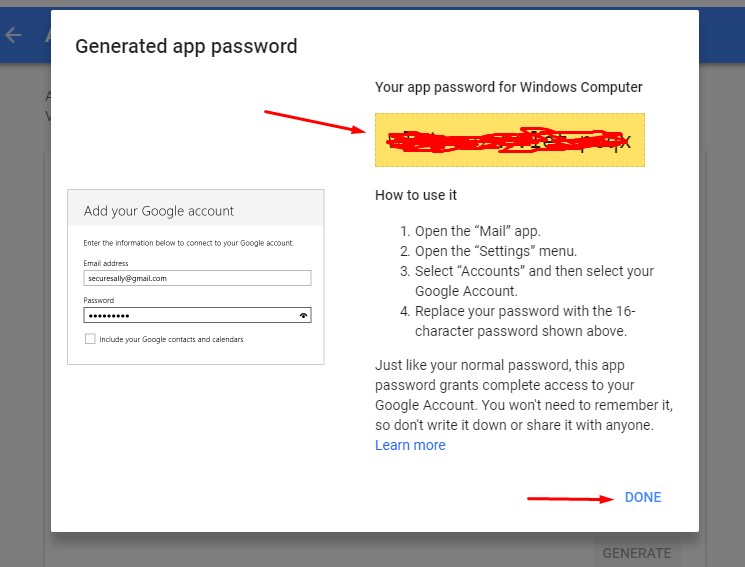
So, Now I've a 16 digit app password.
Second Step:
Go to env file and edit the last part mail options like this,MAIL_DRIVER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=587
MAIL_USERNAME=your_email@gmail.com
MAIL_PASSWORD=your_16_digit_password
MAIL_ENCRYPTION=tls
Ok, now you've setted. Run the config:cache and config:clear command.
php artisan config:cache
php artisan config:clear
Third Step:
For sending email I'll just test this for Contact Page.
Contact Page html Form code is
<form action="{{ route('contact') }}" method="POST">
{{ csrf_field() }}
<div class="form-row">
<div class="form-group col-md-6">
<label for="name" class="col-form-label">Name</label>
<input type="text" class="form-control" id="name" placeholder="your name" name="name" required>
</div>
<div class="form-group col-md-6">
<label for="email" class="col-form-label">Email</label>
<input type="email" class="form-control" id="email" placeholder="Email" name="email" required>
</div>
</div>
<div class="form-group">
<label for="message" class="col-form-label">Message</label>
<textarea class="form-control" id="message" rows="10" name="message" required></textarea>
</div>
<button type="submit" class="btn btn-primary">Send Now</button>
</form>
The output of that HTML form is look like(Using some css extras),
In routes.php or web.php the post contact route is,
Route::post('/contact', 'PageController@sendContact')->name('contact');
Now the Controller sendContact post function is to send a mail in Laravel,
public function sendContact(Request $request){
$this->validate($request, array(
'name' => 'required|max:20',
'email' => 'required|email',
'message' => 'required',
));
$data = array(
'name' =>$request->name,
'email' => $request->email,
'messageBody' => $request->message
);
Mail::send('emails.contact', $data, function($message) use ($data){
$message->from($data['email']);
$message->to('your_email@gmail.com');
$message->subject('Contact Message From Your Site');
});
$request->session()->flash('success', 'Message has sent to admin successfully..!!');
return view('pages.contact');
}
Note: use Mail at top of that class.
use Mail;
Now Look at the Mail::send() function by which Laravel send email to other.
Mail::send('emails.contact', $data, function($message) use ($data){
The function in Mail::send(),
the First parameter 'emails.contact' is the view file, which is in the views/emails/contact.blade.php,
The Second parameter is the $data- the data as an array of the requested user.
The Third is the function including $message as argument
So, we need also an contact.blade.php file which is in the views/emails/ directory,
views/emails/contact.blade.php file is -
<div style="background: #AAAEB4">
<h2 style="text-align: center; background: #4585f4; padding: 25px;">Contact Message From {{ $name }}</h2>
<div style="background: #FFFF99;padding: 30px;">
<h3 style="text-align: center">Contact Message</h3><hr />
<p>Email - {{ $email }}</p>
<div>
{{ $messageBody }}
</div>
</div>
<div style="margin-top: 20px; padding: 10px">
<p style="text-align: center">© All rights reserved <a href="#">PSTU Students</a> - 2017</p>
</div>
</div>
Everythings Done..!!! Now Fill out the form and submit the contact form and look at your inbox of gmail account. In above example, the email format for a specific user is
So, we have completed our email through gmail and if you face any problem, just comment out below, Thanks.
Tags:
How to send Email in Laravel using gmail, Laravel send email, Send Email in Laravel, Send Email to using gmail account laravel.
How to send Email in Laravel using gmail
Reviewed by Maniruzzaman Akash
on
October 14, 2017
Rating:

thanks
ReplyDelete