Send Email Using Gmail in PHP with PHP Mailer -> Every procedure
Hello viewer, today we'll show how to send email using PHP to another email account with gmail.
Let's Start Our Coding..
How to send a simple HTML Mail By PHP using gmail:
First, you can check for everything in PHP Mailer and Skip this full tutorials. Here's the PHPMailer Github Link and documentation -
Github Page - PHPMailer
Gmail example page - Gmail using PHP Mailer
So why from here: To get a clear and concise answer with code, you can use this tutorial.
Step 1:
Download all files from https://github.com/PHPMailer/PHPMailer/tree/master/src and store inside your htdocs or localhost. See the image after storing.
Here Excemption.php, OAuth.php, PHPMailer.php, POP3.php, SMTP.php are the downloaded files from PHP Mailer. and test.php is our main work to send email.
[Here PHPMailer.php and SMTP.php must have to add]
Step 2:
Inside test.php write our code to send a mail from gmail.
First, clear your think about sending mail using gmail.
- You will send an email to other from your email account.
- So, to use this you have to give your gmail email account name and password.
- Then from this email an email will send to other's email account.
test.php code
<?php
// Import PHPMailer classes
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
//Load composer's autoloader
require 'PHPMailer/PHPMailer.php';
require 'PHPMailer/Exception.php';
require 'PHPMailer/SMTP.php';
$mail = new PHPMailer(true); // Passing `true` enables exceptions
try {
//Server settings
$mail->SMTPDebug = 0; // Disable verbose debug output. To enable give 1, 2
$mail->isSMTP(); // Set mailer to use SMTP
$mail->Host = 'smtp.gmail.com';
$mail->SMTPAuth = true;
$mail->Username = 'manirujjamanakash@gmail.com'; // You enter your gamil username
$mail->Password = 'your_gmail_password'; // Gmail password
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
$mail->SMTPOptions = array(
'ssl' => array(
'verify_peer' => false,
'verify_peer_name' => false,
'allow_self_signed' => true
)
);
//Recipients
$mail->setFrom('manirujjamanakash@gmail.com', 'Akash'); // Your gmail username and name
$mail->addAddress('abulkalam.skg@gmail.com', 'Abul Kalam'); // Recioient gmail username and name
//Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
if ($mail->send()) {
echo ('
<div>
<h2 style="text-align:center; background: slateblue; color: white; margin-top: 5% ">Message has sent to senders Email account Successfully</h2>
</div>
');
};
} catch (Exception $e) {
echo 'Message could not be sent.';
echo 'Mailer Error: ' . $mail->ErrorInfo;
}
[Clear thing:]
Here my gmail is manirujjamanakash@gmail.com and so, I've used it. You will enter your gmail username.
Then enter recipient gmail -abulkalam.skg@gmail.com. You will enter your expected recipient email.
Output in the recipients email account:
That's how We've sent a gmail account to another gmail or email account.
Download Complete Code With Files
Download Complete Code With Files
If Any Error occur:
That means, in your gmail, you haven't allow the less secure apps. To allow this,
- Goto your gmail account
- Goto Sign in and Security Page and allow the Less Secure apps.
Now Try and I hope you can successfully send Email Using gmail.
If You have two step verification in gmail:
If you have two step verification, then you may use password for your web apps. By generating a new password for web apps. See the procedure to generate a password in gmail.
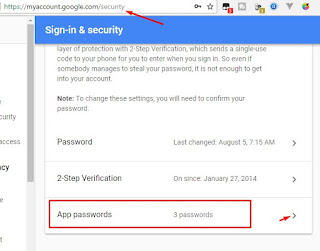
Now select the boxes and set Mail and Windows Computer or any and click on generate.
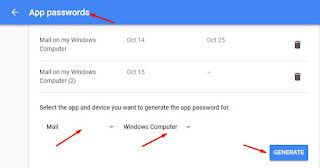
After clicking on Generate, you will get a password like
Now you can use every where this password, instead of your real password. That's everything how you can send email using your gmail account with PHP.
Now if you want to add multiple things in your Email, just Use PHPMailer Example pages source codes for gmail.
Demo Code:
<?php
//Import PHPMailer classes into the global namespace
use PHPMailer\PHPMailer\PHPMailer;
require '../vendor/autoload.php';
//Create a new PHPMailer instance
$mail = new PHPMailer;
//Tell PHPMailer to use SMTP
$mail->isSMTP();
//Enable SMTP debugging
// 0 = off (for production use)
// 1 = client messages
// 2 = client and server messages
$mail->SMTPDebug = 2;
//Set the hostname of the mail server
$mail->Host = 'smtp.gmail.com';
// use
// $mail->Host = gethostbyname('smtp.gmail.com');
// if your network does not support SMTP over IPv6
//Set the SMTP port number - 587 for authenticated TLS, a.k.a. RFC4409 SMTP submission
$mail->Port = 587;
//Set the encryption system to use - ssl (deprecated) or tls
$mail->SMTPSecure = 'tls';
//Whether to use SMTP authentication
$mail->SMTPAuth = true;
//Username to use for SMTP authentication - use full email address for gmail
$mail->Username = "username@gmail.com";
//Password to use for SMTP authentication
$mail->Password = "yourpassword";
//Set who the message is to be sent from
$mail->setFrom('from@example.com', 'First Last');
//Set an alternative reply-to address
$mail->addReplyTo('replyto@example.com', 'First Last');
//Set who the message is to be sent to
$mail->addAddress('whoto@example.com', 'John Doe');
//Set the subject line
$mail->Subject = 'PHPMailer GMail SMTP test';
//Read an HTML message body from an external file, convert referenced images to embedded,
//convert HTML into a basic plain-text alternative body
$mail->msgHTML(file_get_contents('contents.html'), __DIR__);
//Replace the plain text body with one created manually
$mail->AltBody = 'This is a plain-text message body';
//Attach an image file
$mail->addAttachment('images/phpmailer_mini.png');
//send the message, check for errors
if (!$mail->send()) {
echo "Mailer Error: " . $mail->ErrorInfo;
} else {
echo "Message sent!";
}
[Having any problem or further issues, just comment here.]
Tags: Send Email Using Gmail in PHP with PHP Mailer -> Every procedure, Gmail with php, Send email with PHP, Send email wit gmail
Send Email Using Gmail in PHP with PHP Mailer -> Every procedure
Reviewed by Maniruzzaman Akash
on
December 09, 2017
Rating:

izmir
ReplyDeleteErzurum
Diyarbakır
Tekirdağ
Ankara
2XP
ankara parça eşya taşıma
ReplyDeletetakipçi satın al
antalya rent a car
antalya rent a car
ankara parça eşya taşıma
C4PS5
02724
ReplyDeleteMersin Evden Eve Nakliyat
Antalya Evden Eve Nakliyat
Bursa Lojistik
Şırnak Parça Eşya Taşıma
Karabük Evden Eve Nakliyat
AECA8
ReplyDeletebinance referans
BAC25
ReplyDeletebinance %20 referans kodu
257F4
ReplyDeletehatay parasız sohbet siteleri
kayseri sohbet chat
osmaniye görüntülü sohbet siteleri
mobil sohbet chat
görüntülü sohbet
sinop görüntülü sohbet yabancı
samsun ücretsiz görüntülü sohbet
bayburt en iyi sesli sohbet uygulamaları
sohbet uygulamaları
1EA96
ReplyDeleteısparta canlı ücretsiz sohbet
batman en iyi görüntülü sohbet uygulamaları
yabancı görüntülü sohbet siteleri
ısparta Tamamen Ücretsiz Sohbet Siteleri
nevşehir bedava görüntülü sohbet sitesi
sohbet sitesi
en iyi görüntülü sohbet uygulamaları
Bilecik Canlı Görüntülü Sohbet Odaları
kayseri bedava sohbet odaları
C28DC
ReplyDeleteücretsiz sohbet odaları
Kastamonu Ücretsiz Sohbet Uygulaması
bedava sohbet siteleri
ağrı en iyi ücretsiz görüntülü sohbet siteleri
burdur telefonda sohbet
nanytoo sohbet
ankara sesli sohbet uygulamaları
sohbet muhabbet
Kocaeli Kadınlarla Sohbet Et
034FB
ReplyDeleteCoin Çıkarma
Bitcoin Kazma Siteleri
Youtube İzlenme Hilesi
Pepecoin Coin Hangi Borsada
Tiktok İzlenme Hilesi
Clubhouse Takipçi Satın Al
Binance Referans Kodu
Paribu Borsası Güvenilir mi
Alyattes Coin Hangi Borsada
29751
ReplyDeleteBinance Referans Kodu
Instagram Beğeni Satın Al
Binance Hesap Açma
Binance Sahibi Kim
Likee App Beğeni Satın Al
Bitcoin Nasıl Üretilir
Arbitrum Coin Hangi Borsada
Bitcoin Mining Nasıl Yapılır
Binance Komisyon Ne Kadar